Harness the full potential of Snowflake scripting to streamline data operations and automate complex business logics. Dive into this practical guide to discover how scripting blocks transform your Snowflake scripting experience, enabling:
- Precise control over data flows
- Optimized conditional logic
- Efficient looping structures
- Custom error handling
- And more!
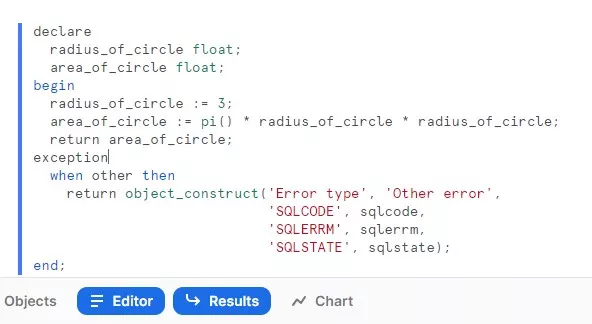
Page Content
Understanding Blocks in Snowflake Scripting
In Snowflake Scripting, blocks serve as essential building blocks for organizing and executing procedural code. Similar to other database programming language, blocks are defined using specific keywords to structure your code into different sections, each with a clear purpose.
Structure of Block in a Snowflake Scripting
Snowflake scripting block has the following basic structure:
DECLARE
... variable declarations, ...
... cursor declarations, etc ...
BEGIN
... Snowflake Scripting and SQL statements ...
EXCEPTION
... statements for handling exceptions ...
END;
Here’s a breakdown of the key components of a block:
- DECLARE: This optional section is where you declare variables, cursors, result sets, and custom exceptions that will be used within the block.
- BEGIN…END: This mandatory section is the main body of your procedural code. It’s where you’ll write SQL statements, control flow structures (like loops and conditionals), and other procedural logic.
- EXCEPTION: This optional section is allows you to handling errors that may arise during code execution. It allows you to efficiently trap exceptions and perform appropriate actions to address them.
By effectively utilizing blocks, you can achieve the following:
- Structured Code: Organize your code into different logical groups, making it easier to read, understand, and maintain.
- Control Flow: Implement conditional logic and looping structures to handle different scenarios and automate repetitive tasks.
- Error Handling: Gracefully handle potential errors and prevent your script from failing unexpectedly.
- Modularization: Break down complex tasks into smaller, reusable blocks to enhance code reusability and maintainability.
Types of Blocks in Snowflake Scripting
Within Snowflake Scripting, blocks come in two different forms, each designed for specific use cases
Stored Procedure Blocks
A stored procedure serves as a container for encapsulating business logic, capable of managing complex operations like branching and looping structures, return results, gracefully handle errors, and even create dynamic SQL statements within stored procedures.
The Snowflake scripting stored procedure comprises distinct sections, including DECLARE
, BEGIN
, EXCEPTION
, and END
, organizing the code’s structure and functionality.
The following is an example that uses various blocks:
create or replace procedure procedure_blocks()
returns float
language sql
as
declare
radius float;
area_of_circle float;
begin
radius := 3;
area_of_circle := pi() * radius * radius;
return area_of_circle;
exception
when other then
return object_construct('Error type', 'Other error',
'SQLCODE', sqlcode,
'SQLERRM', sqlerrm,
'SQLSTATE', sqlstate);
end;
Anonymous Blocks in Snowflake Script
In Snowflake Scripting, anonymous blocks offer a flexible means to execute procedural code without the need to create a formal stored procedure. They reside outside the confines of stored procedures, providing a distinct execution model.
Anonymous blocks are defined as self-contained SQL statements, allowing you to execute them directly within a session or script. Unlike stored procedures, they aren’t persistently stored in the database. Instead, they exist only for the duration of the session or script in which they’re executed.
The following is an example of an anonymous block:
declare
radius_of_circle float;
area_of_circle float;
begin
radius_of_circle := 3;
area_of_circle := pi() * radius_of_circle * radius_of_circle;
return area_of_circle;
exception
when other then
return object_construct('Error type', 'Other error',
'SQLCODE', sqlcode,
'SQLERRM', sqlerrm,
'SQLSTATE', sqlstate);
end;
The BEGIN and END block that defines the block will also executes the block. You don’t have to call separate CALL statement to execute this block.
Common Snowflake Block Use Cases
Following are some of the Snowflake script block use cases:
- Data Manipulation:
- Creating tables and loading data using DDL statements within blocks.
- Updating and deleting data with conditional checks inside blocks.
- Branching Logic:
- Error Handling:
- Using the EXCEPTION block to trap and handle errors easily.
- Logging error messages and taking corrective actions within blocks.
Advanced Snowflake Block Use Cases
Following are the advance use cases of Snowflake scripting blocks:
- Return Resultset:
- Returning values from blocks using the RETURN statement.
- Return result set in the form of table.
- Use stored procedure and user-defined functions within the Snowflake scripting blocks.
Conclusion
In conclusion, Snowflake scripting Stored procedures blocks are ideal for complex, reusable logic, offering organization and persistence. and Snowflake scripting anonymous blocks are perfect for on-demand execution, testing, and one-time tasks, prioritizing flexibility.
Related Articles,
- How to Create Synonyms in Snowflake – Alternate Method
- How to Get Rows Affected by Last Snowflake SQL Query?
- How to Handle NULL in Snowflake? Functions
Hope this helps 🙂